For now, this guide will be for Windows specifically. Later on it will list alternative Unix-style commands (e.g. for WSL, Ubuntu, and macOS).
If within your Node project directory you do npm list @wdio/cli
, what’s the first number after -- @wdio/cli@
in the output? If it’s 6, see
the instructions for WebdriverIO 6 below. If it’s 5, see the instructions for WebdriverIO 5 below.
WebdriverIO 6
WebdriverIO 6 bundles its own native assertion library, expect-webdriverio
.
Configure expect-webdriverio
The only thing you have to do to activate it is the following.
- Open up your
wdio.conf.js
in your IDE - Uncomment the
beforeSession
section - Add
require('expect-webdriverio')
So it becomes:
beforeSession: function (config, capabilities, specs) {
require('expect-webdriverio')
},
That’s it. You don’t need to call it within test files, it will get called at the start of each session.
Write a test
Let’s start as simply as we possibly can. For my examples I will use https://www.50five.nl as my test page.
describe('the 50five homepage', () => {
it('should have a pending test', () => {
})
it('should include 50five in the page title', () => {
browser.url('/')
expect(browser).toHaveTitle('50five', { containing: true })
})
})
Translation:
- Browser object, go to the
baseUrl
at its root. - Tell me whether the value in the
<title>
tag of the current browser tab contains the substring50five
.
Run npm t
. The result should be something like:
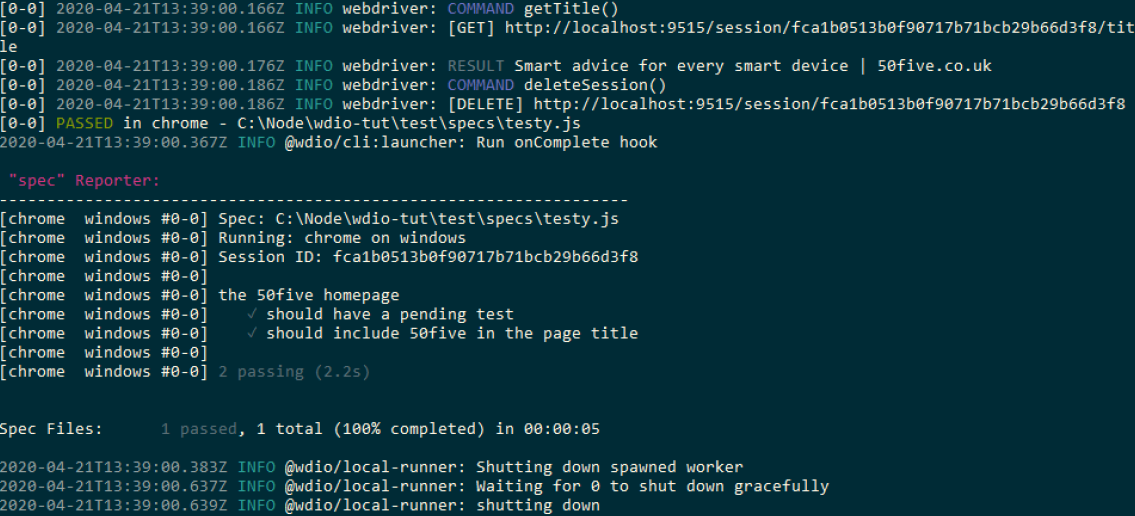
WebdriverIO 5
By default, WebdriverIO 5 uses Mocha's assertion library. Mocha's default assertion module only supports the functions listed on the Node.js Assertion Testing page, which is very limited. We will definitely want to change this, so we can assert in more ways and more elegantly.
A nice way to do this is with Chai’s ‘expect’ assertions.
Install Chai
We’ll need to install the Mocha adapter for WebdriverIO, and then the Chai package. Make sure you’re in your project folder, then run:
npm i --save-dev @wdio/mocha-framework
And:
npm i --save-dev chai
Now let’s get it configured.
Configure Chai
In your IDE, open up wdio.conf.js
.
Find this commented out section:
// before: function (capabilities, specs) {
// },
Uncomment it, so it becomes:
before: function (capabilities, specs) {
},
As it explains right above that section, this gets executed before test execution begins. There are also other commented out sections where you could define what should run before each test, after each test, or what should run before a session is initialised, etc. We won’t bother with those now.
Now, let’s call Chai in this before
section:
before: function (capabilities, specs) {
var chai = require('chai');
global.expect = chai.expect;
chai.Should();
},
Save the file.
Now open up your test file. If you’re following on from Working with WebdriverIO | Basic setup, this will be tutorial.js
and it will contain:
const assert = require('assert');
describe('the 50five homepage', () => {
it('should have a pending test', () => {
})
})
Change this to:
var expect = require('chai').expect
describe('the 50five homepage', () => {
it('should have a pending test', () => {
})
})
This is still an empty test, but we want to know Chai is working right before trying anything else:
npm t
The output should still be 1 passing test.
Write a test
Okay, now we can get to business.
Let’s start as simply as we possibly can:
var expect = require('chai').expect
describe('the 50five homepage', () => {
it('should have a pending test', () => {
})
it('should include 50five in the page title', () => {
browser.url('/')
expect(browser.getTitle()).to.include('50five')
})
})
Translation:
- Browser object, go to the
baseUrl
at its root. - Browser object, get the value in the
<title>
tag - Tell me whether the value in the
<title>
tag contains the substring50five
.
Run npm t
. The result should be something like:
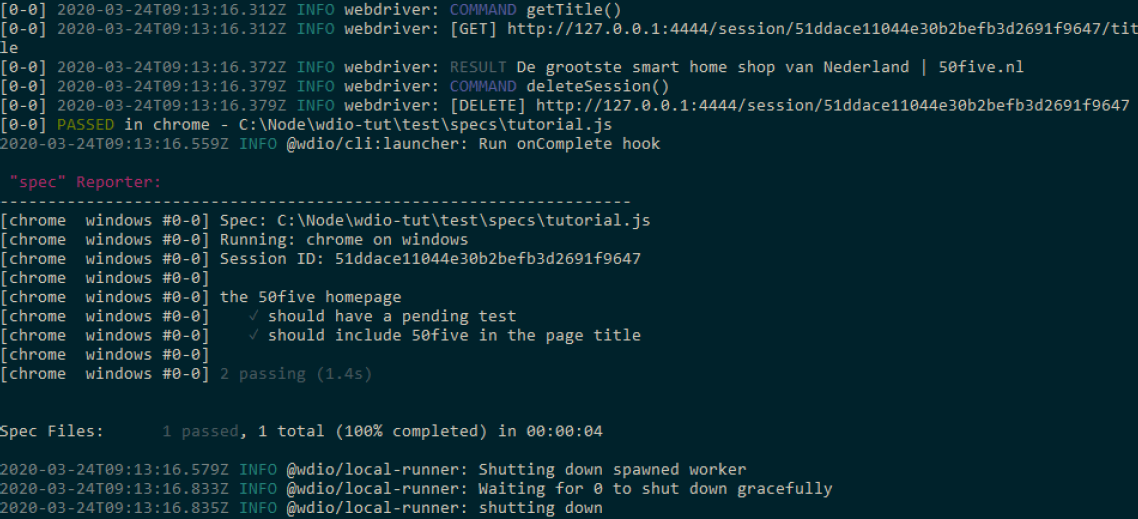
Next steps
That’s the first real test done!
Is it a useful test? Not really. We’ll work on that.
In other articles we’ll look at:
- How do you test interesting things like login, search, interacting with overlays?
- How do you switch from Chai to using Cucumber, if so desired?
- How do you debug problematic tests?
- How do you determine what to test and how to test, what are common pitfalls and solutions?
- How do you tell a bad test from a good test?
- How do you create a repo for the tests and work with that repo?